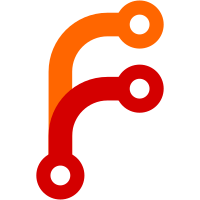
git-svn-id: svn://svn.cy55.de/Zope3/src/loops/trunk@2558 fd906abe-77d9-0310-91a1-e0d9ade77398
167 lines
5.6 KiB
Text
167 lines
5.6 KiB
Text
===============================================================
|
|
loops - Linked Objects for Organization and Processing Services
|
|
===============================================================
|
|
|
|
($Id$)
|
|
|
|
>>> from zope import component
|
|
>>> from zope.traversing.api import getName
|
|
|
|
First we set up a loops site with basic and example concepts and resources.
|
|
|
|
>>> from zope.app.testing.setup import placefulSetUp, placefulTearDown
|
|
>>> site = placefulSetUp(True)
|
|
|
|
>>> from loops.organize.setup import SetupManager
|
|
>>> component.provideAdapter(SetupManager, name='organize')
|
|
>>> from loops.tests.setup import TestSite
|
|
>>> t = TestSite(site)
|
|
>>> concepts, resources, views = t.setup()
|
|
>>> loopsRoot = site['loops']
|
|
|
|
|
|
Stateful Objects
|
|
================
|
|
|
|
A simple publishing workflow
|
|
----------------------------
|
|
|
|
Let's start with registering the states definitions and adapters needed.
|
|
The states definition (aka 'workflow') is registered as a utility; for
|
|
making an object statful we'll use an adapter.
|
|
|
|
>>> from cybertools.stateful.interfaces import IStatesDefinition, IStateful
|
|
>>> from cybertools.stateful.publishing import simplePublishing
|
|
>>> component.provideUtility(simplePublishing(), name='loops.simple_publishing')
|
|
|
|
>>> from loops.organize.stateful.base import SimplePublishable
|
|
>>> component.provideAdapter(SimplePublishable, name='loops.simple_publishing')
|
|
|
|
We may now take a document and adapt it to IStateful so that we may
|
|
check the document's state and perform transitions to other states.
|
|
|
|
>>> doc01 = resources['d001.txt']
|
|
>>> statefulDoc01 = component.getAdapter(doc01, IStateful,
|
|
... name='loops.simple_publishing')
|
|
|
|
>>> statefulDoc01.state
|
|
'draft'
|
|
|
|
>>> statefulDoc01.doTransition('publish')
|
|
>>> statefulDoc01.state
|
|
'published'
|
|
|
|
Let's check if the state is really stored in the underlying object and
|
|
not just kept in the adapter.
|
|
|
|
>>> statefulDoc01_x = component.getAdapter(doc01, IStateful,
|
|
... name='loops.simple_publishing')
|
|
|
|
>>> statefulDoc01.state
|
|
'published'
|
|
|
|
|
|
Controlling classification quality
|
|
----------------------------------
|
|
|
|
We again first have to register states definitions and adapter classes.
|
|
|
|
>>> from loops.organize.stateful.quality import classificationQuality
|
|
>>> component.provideUtility(classificationQuality(),
|
|
... name='loops.classification_quality')
|
|
>>> from loops.organize.stateful.quality import ClassificationQualityCheckable
|
|
>>> component.provideAdapter(ClassificationQualityCheckable,
|
|
... name='loops.classification_quality')
|
|
>>> from loops.organize.stateful.quality import assign, deassign
|
|
>>> component.provideHandler(assign)
|
|
>>> component.provideHandler(deassign)
|
|
|
|
Now we can get a stateful adapter for a resource.
|
|
|
|
>>> qcheckedDoc01 = component.getAdapter(doc01, IStateful,
|
|
... name='loops.classification_quality')
|
|
>>> qcheckedDoc01.state
|
|
'new'
|
|
|
|
Let's create two customer objects to be used for classification of resources
|
|
later.
|
|
|
|
>>> tCustomer = concepts['customer']
|
|
>>> from loops.concept import Concept
|
|
>>> from loops.setup import addAndConfigureObject
|
|
>>> c01 = addAndConfigureObject(concepts, Concept, 'c01', conceptType=tCustomer,
|
|
... title='im publishing')
|
|
>>> c02 = addAndConfigureObject(concepts, Concept, 'c02', conceptType=tCustomer,
|
|
... title='DocFive')
|
|
|
|
When we change the concept assignments of the resource - i.e. its classification
|
|
- the classification quality state changes automaticalls
|
|
|
|
>>> c01.assignResource(doc01)
|
|
>>> qcheckedDoc01 = component.getAdapter(doc01, IStateful,
|
|
... name='loops.classification_quality')
|
|
>>> qcheckedDoc01.state
|
|
'classified'
|
|
|
|
>>> c02.assignResource(doc01)
|
|
>>> qcheckedDoc01.state
|
|
'classified'
|
|
|
|
In order to mark the classification as "verified" (i.e. quality-checked)
|
|
we have to perform the corresponding transition explicitly.
|
|
|
|
>>> qcheckedDoc01.doTransition('verify')
|
|
>>> qcheckedDoc01.state
|
|
'verified'
|
|
|
|
Upon later changes of classification the "verified" state gets lost again.
|
|
|
|
>>> c02.deassignResource(doc01)
|
|
>>> qcheckedDoc01.state
|
|
'classified'
|
|
|
|
>>> c01.deassignResource(doc01)
|
|
>>> qcheckedDoc01.state
|
|
'unclassified'
|
|
|
|
Changing states when editing
|
|
----------------------------
|
|
|
|
We first need a node that provides us access to the resource as its target
|
|
|
|
>>> from loops.view import Node
|
|
>>> node = addAndConfigureObject(views, Node, 'node', target=doc01)
|
|
|
|
>>> from loops.browser.form import EditObjectForm, EditObject
|
|
>>> from zope.publisher.browser import TestRequest
|
|
|
|
The form view gives us access to the states of the object.
|
|
|
|
>>> loopsRoot.options = ['organize.stateful.resource:'
|
|
... 'loops.classification_quality,loops.simple_publishing']
|
|
|
|
>>> form = EditObjectForm(node, TestRequest())
|
|
>>> for st in form.states:
|
|
... sto = st.getStateObject()
|
|
... transitions = st.getAvailableTransitions()
|
|
... userTrans = st.getAvailableTransitionsForUser()
|
|
... print st.statesDefinition, sto.title, [t.title for t in transitions],
|
|
... print [t.title for t in userTrans]
|
|
loops.classification_quality unclassified ['classify', 'verify'] ['verify']
|
|
loops.simple_publishing published ['retract', 'archive'] ['retract', 'archive']
|
|
|
|
Let's now update the form.
|
|
|
|
>>> input = {'state.loops.classification_quality': 'verify'}
|
|
>>> proc = EditObject(form, TestRequest(form=input))
|
|
>>> proc.update()
|
|
False
|
|
|
|
>>> qcheckedDoc01.state
|
|
'verified'
|
|
|
|
|
|
Fin de partie
|
|
=============
|
|
|
|
>>> placefulTearDown()
|